Note
Go to the end to download the full example code.
Postprocess a solid model#
This example shows features which are tailored for the postprocessing of solid models. These are
Failure plot on the reference surface
Sampling point for solid elements
The model is an assembly with solid and shell elements. So, the example also demonstrates how to distinguish between different element types.
Note
When using a Workbench project,
use the get_composite_files_from_workbench_result_folder()
method to obtain the input files.
Set up analysis#
Setting up the analysis consists of loading Ansys libraries, connecting to the DPF server, and retrieving the example files.
Load Ansys libraries.
from ansys.dpf.composites.composite_model import CompositeModel
from ansys.dpf.composites.constants import FailureOutput
from ansys.dpf.composites.example_helper import get_continuous_fiber_example_files
from ansys.dpf.composites.failure_criteria import (
CombinedFailureCriterion,
CoreFailureCriterion,
FaceSheetWrinklingCriterion,
HashinCriterion,
MaxStressCriterion,
)
from ansys.dpf.composites.layup_info import SolidStackProvider
from ansys.dpf.composites.result_definition import FailureMeasureEnum
from ansys.dpf.composites.server_helpers import connect_to_or_start_server
from ansys.dpf.composites.solid_stack_results import get_through_the_thickness_results
# Start a DPF server and copy the example files into the current working directory.
server = connect_to_or_start_server()
composite_files_on_server = get_continuous_fiber_example_files(server, "assembly")
Configure combined failure criterion#
Configure the combined failure criterion.
combined_fc = CombinedFailureCriterion(
name="failure of all materials",
failure_criteria=[
MaxStressCriterion(),
CoreFailureCriterion(),
FaceSheetWrinklingCriterion(),
HashinCriterion(),
],
)
Set up model#
Set up the composite model.
composite_model = CompositeModel(composite_files_on_server, server)
Plot failure results on reference surface#
The first feature is to plot the failure results on the reference surface. This feature projects the overall maximum failure value and according failure model from the solid elements to the shell elements. This makes the critical areas of the solid elements visible on the reference surface.
output_all_elements = composite_model.evaluate_failure_criteria(
combined_criterion=combined_fc,
)
irf_on_ref_surface_field = output_all_elements.get_field(
{"failure_label": FailureOutput.FAILURE_VALUE_REF_SURFACE}
)
irf_on_ref_surface_field.plot()
# The next plot shows the same failure analysis without the projection
# to the reference surface for the sake of comparison.
irf_max_field = output_all_elements.get_field({"failure_label": FailureOutput.FAILURE_VALUE})
irf_max_field.plot()
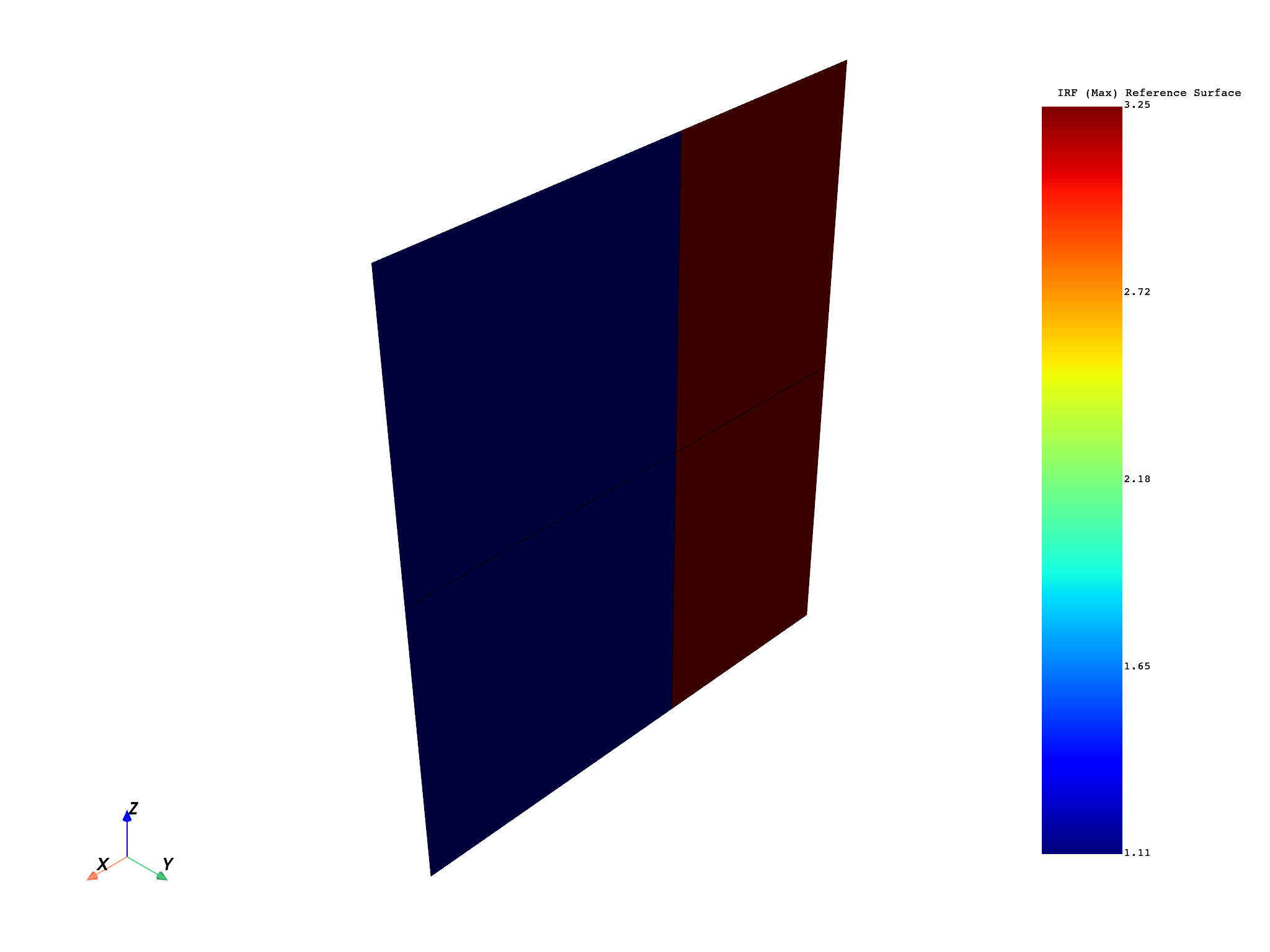
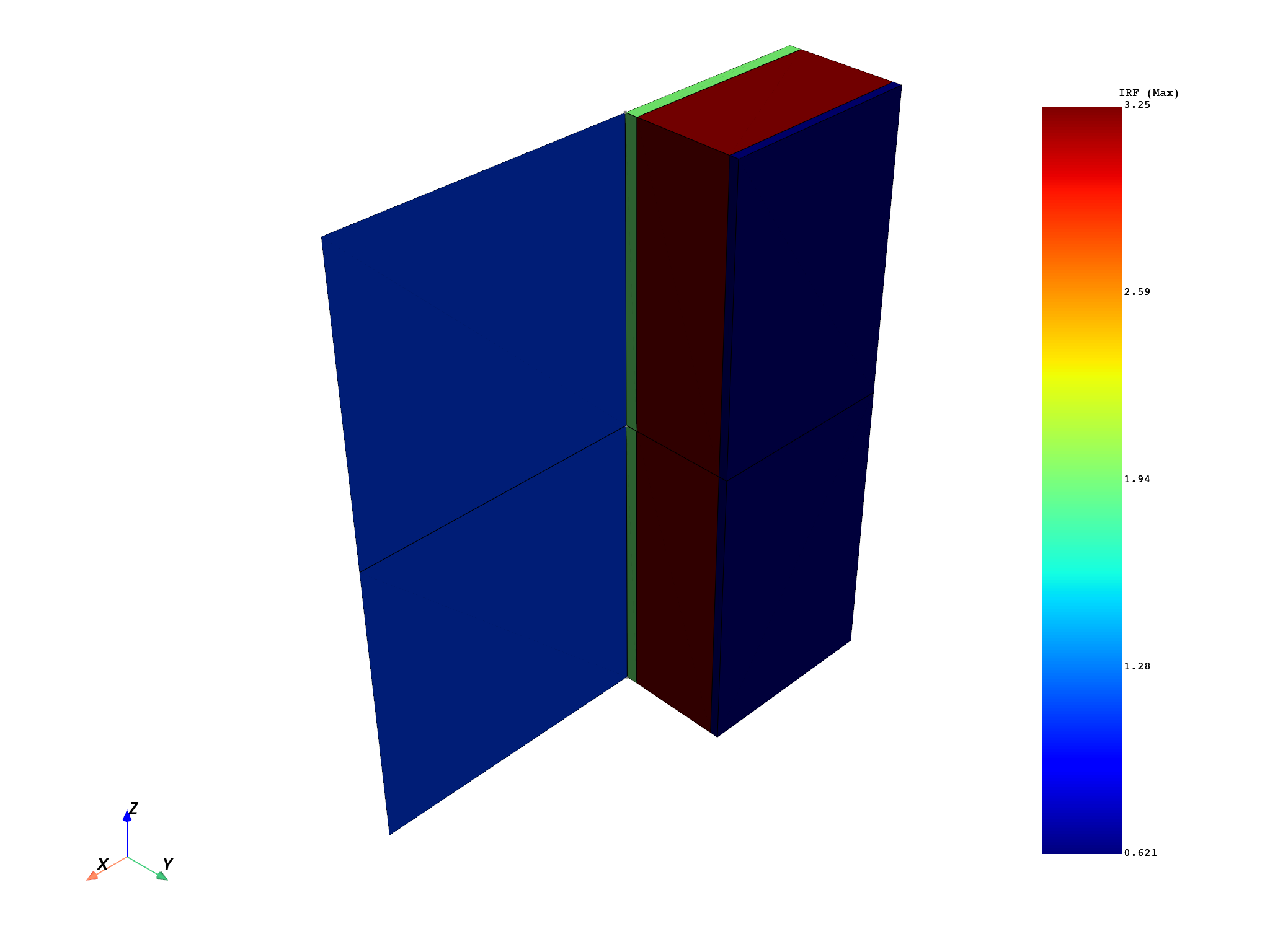
Sampling point for solids#
The next feature is to create a sampling point for solid elements. The user selects one solid element and the feature automatically selects the entire stack of solid elements of the laminate to plot the through-the-thickness results. So, the first step is to find the critical solid element(s). In this example, the one with the highest IRF is selected.
critical_solid_id = 0
critical_irf = -1.0
critical_solid_elements = {}
for index, element_id in enumerate(irf_max_field.scoping.ids):
# only consider solid elements because the model is an assembly of shells and solids
if not composite_model.get_element_info(element_id).is_shell:
irf = irf_max_field.get_entity_data(index)[0]
if irf > critical_irf:
critical_solid_id = element_id
critical_irf = irf
sampling_point_solid_stack = composite_model.get_sampling_point(
combined_criterion=combined_fc, element_id=critical_solid_id
)
The plot shows the lay-up of the solid stack, stresses and failure results. The solid elements are indicated in the layup plot by the colored boxes. In this case, the first solid element has 3 layers, the second has 1 layer (core), and the third has 2 layers.
core_scale_factor = 0.2
sampling_point_plot = sampling_point_solid_stack.get_result_plots(
strain_components=(), # Skip strains
core_scale_factor=core_scale_factor,
failure_components=(FailureMeasureEnum.INVERSE_RESERVE_FACTOR,),
show_failure_modes=True,
)
sampling_point_plot.figure.set_figheight(8)
sampling_point_plot.figure.set_figwidth(12)
sampling_point_plot.figure.show()
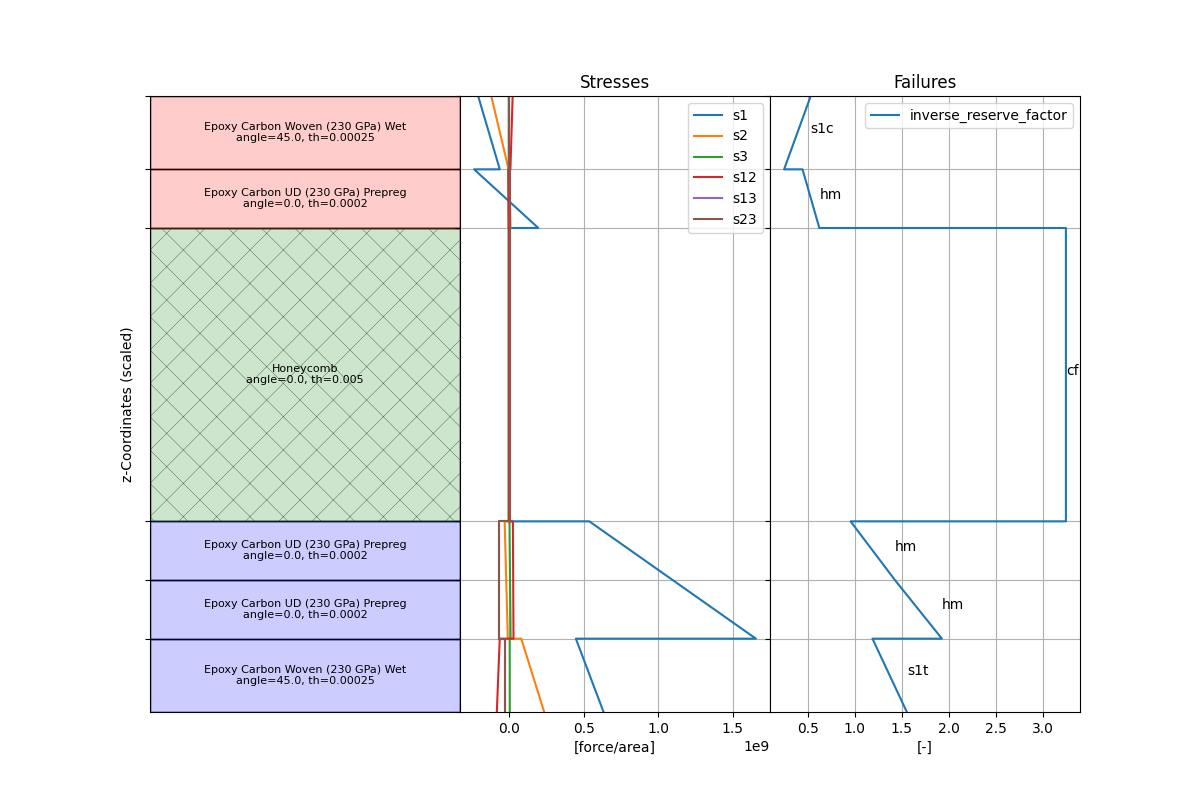
Here is another sampling point plot with the in-plane strains
sampling_point_plot = sampling_point_solid_stack.get_result_plots(
strain_components=("e1", "e2", "e12"), # Show in-plane results only
stress_components=(), # Don't show stresses
failure_components=(),
core_scale_factor=core_scale_factor,
create_laminate_plot=False,
show_failure_modes=True,
)
sampling_point_plot.figure.set_figheight(8)
sampling_point_plot.figure.set_figwidth(12)
sampling_point_plot.figure.show()
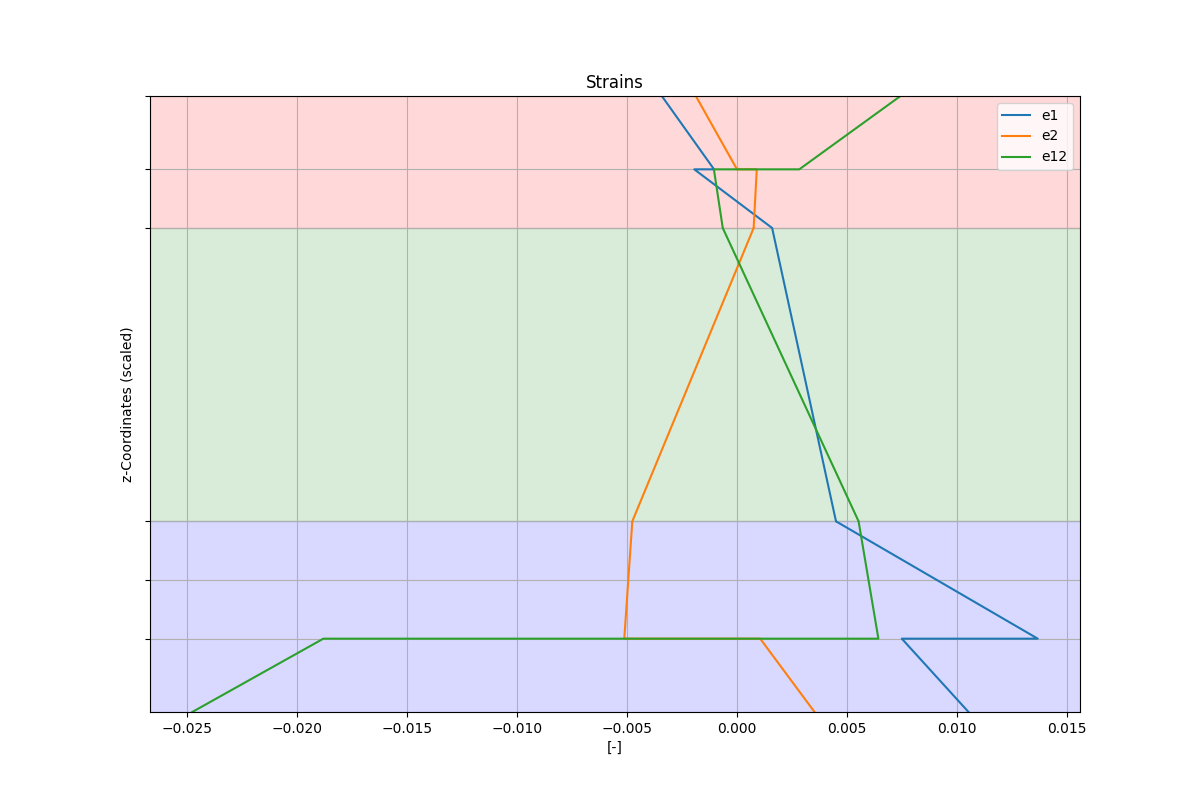
Solid Stack Information#
Information about the stack of solid elements can be retrieved by using the SolidStackProvider. A basic example is shown below where 6 is the element ID (label) and not an index.
solid_stack_provider = SolidStackProvider(
composite_model.get_mesh(), composite_model.get_layup_operator()
)
print(f"Number of solid stacks: {solid_stack_provider.number_of_stacks}")
stack_of_element_6 = solid_stack_provider.get_solid_stack(6)
print(f"Solid stack of element 6: {stack_of_element_6}")
Number of solid stacks: 2
Solid stack of element 6: SolidStack(element_ids=[np.int32(6), np.int32(8), np.int32(10)], element_wise_analysis_plies={np.int32(6): ['solid::P1L1__woven_45', 'solid::P1L1__ud_patch ns1', 'solid::P1L1__ud'], np.int32(8): ['solid::P1L1__core'], np.int32(10): ['solid::P1L1__ud.2', 'solid::P1L1__woven_45.2']}, element_ply_thicknesses={6: [0.00025, 0.0002, 0.0002], 8: [0.005000000000000001], 10: [0.00019999999999999996, 0.00024999999999999995]}, element_wise_levels={6: np.int32(0), 8: np.int32(1), 10: np.int32(2)})
The solid stack can then be used for example to get the through-the-thickness results. In this example, s11, s22 and s12 are retrieved for the last time step.
stress_operator = composite_model.core_model.results.stress()
stress_operator.inputs.bool_rotate_to_global(False)
stress_container = stress_operator.outputs.fields_container()
stress_field = stress_container.get_field(
{
"time": stress_container.get_available_ids_for_label("time")[-1],
}
)
results = get_through_the_thickness_results(
solid_stack=stack_of_element_6,
element_info_provider=composite_model.get_element_info_provider(),
result_field=stress_field,
component_names=("s1", "s2", "s12"),
)
print(results)
{'s1': [236009100.0, 82238220.0, 1655682496.0, 1097056512.0, 1097056512.0, 538430544.0, -2590.4479217529297, -2853.4053344726562, 196753936.0, -234099612.0, -2539490.375, -118968348.0], 's2': [634072800.0, 448304904.0, -7902233.0, -18554689.5, -18554689.5, -29207148.25, -8803.749724388123, -3417.094207763672, 10060274.8125, 2203868.5, -61806631.0, -206908704.0], 's12': [-81675167.0, -62011951.0, -30245672.5, -28130711.0, -28130711.0, -26015750.0, -0.005535265867365524, 0.0006386361201293766, 3001589.77734375, 4896852.3125, 9372089.75, 24551776.0]}
Total running time of the script: (0 minutes 14.782 seconds)